Bundle JavaScript
Prepros can bundle your JavaScript with Webpack. Just enable “Bundle Imports and Requires” option in the file options sidebar. Prepros also transpiles all imported JavaScript files before bundling them if you enable the “Transpile with Babel” option.
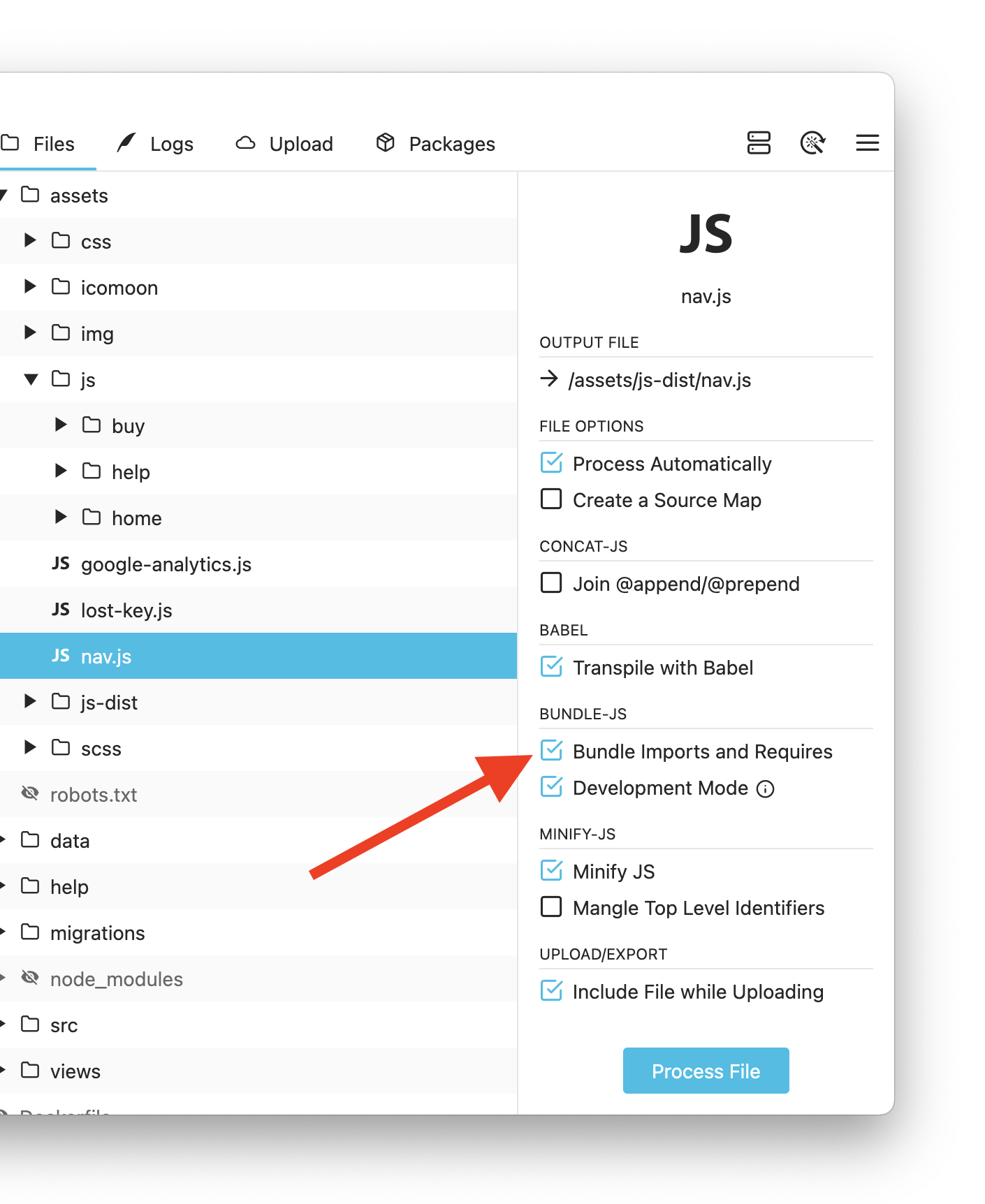
You can import files with both ES6 import syntax and commonjs require function.
import React from 'react'; // imports this from node_modules
import './file.js';
require('./another.js');
You can also import modules from node_modules folder after installing them with the built in npm package manager
Global Variables
If you want to substitute global variables in your output bundle, you can go to Project Settings -> JS Tools -> Bundle then use the “Global Variables” option.
if (DEBUG) {
console.log('This is Debug Mode');
}
When you set DEBUG
global to true
.
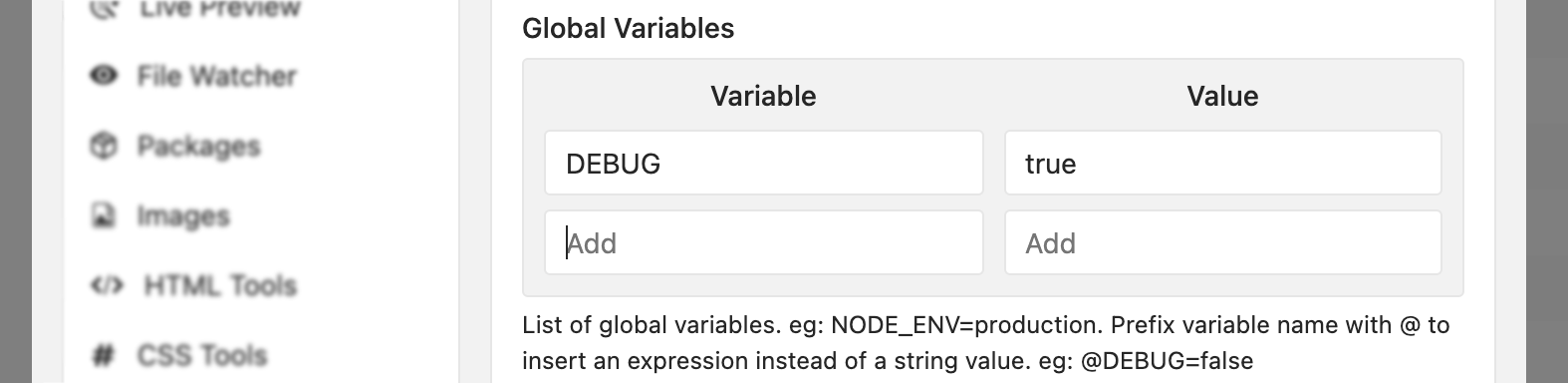
The output will be as follows.
if ('true') {
console.log('This is Debug Mode');
}
This is probably not what you expect, Prepros substitutes global variables as strings by default but if you want to substitute a boolean or an expression you can prefix the variable name with @
character to substitute an expression. So you should set @DEBUG
to true
to substitute DEBUG
global variable with the boolean value true
.
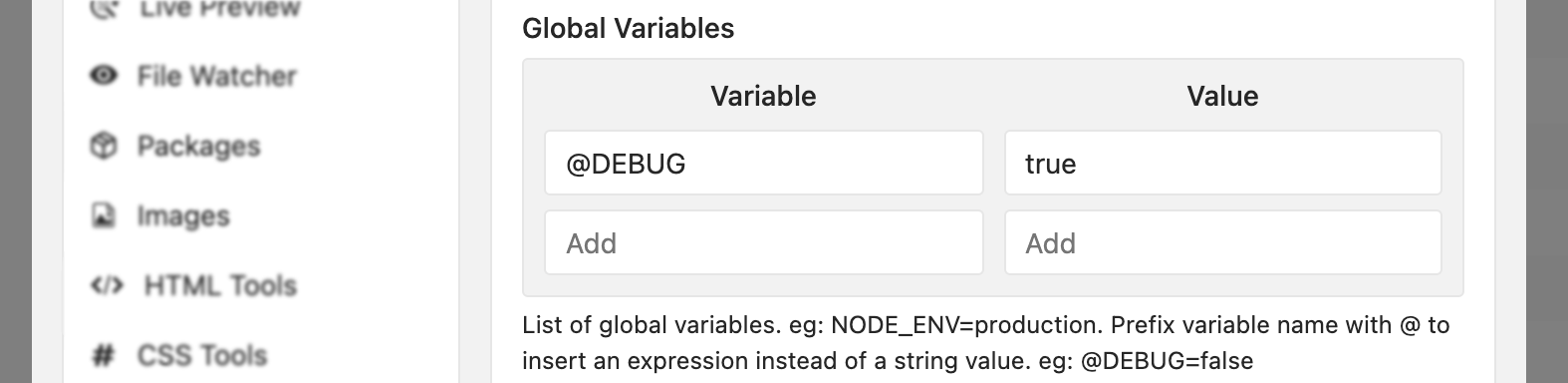
Then the output will be as follows.
if (true) {
console.log('This is Debug Mode');
}
External Modules
If you have already added a library or module to your html page with a script tag. Eg: React from cdnjs.org or jQuery from jQuery CDN, You can mark a module as external from Project Settings -> JS Tools -> Bundle to prevent it from being bundled from node_modules folder.
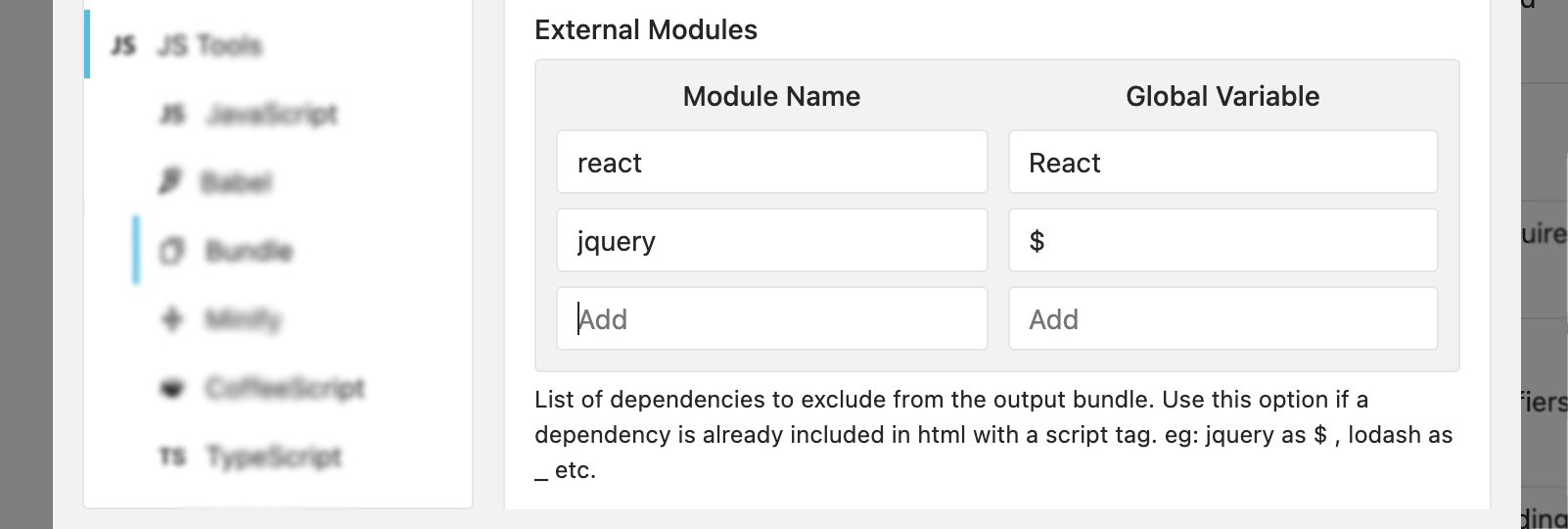
import React from 'react';
import $ from 'jquery';
In this case both react
and jquery
are not bundled from node_modules folder. They are loaded from window.React
and window.$
when the output file is used.
Legacy @append/@prepend Syntax
You can enable support for legacy “@append/@prepend” statements by enabling the “Join @append/@prepend” option in the file options sidebar.
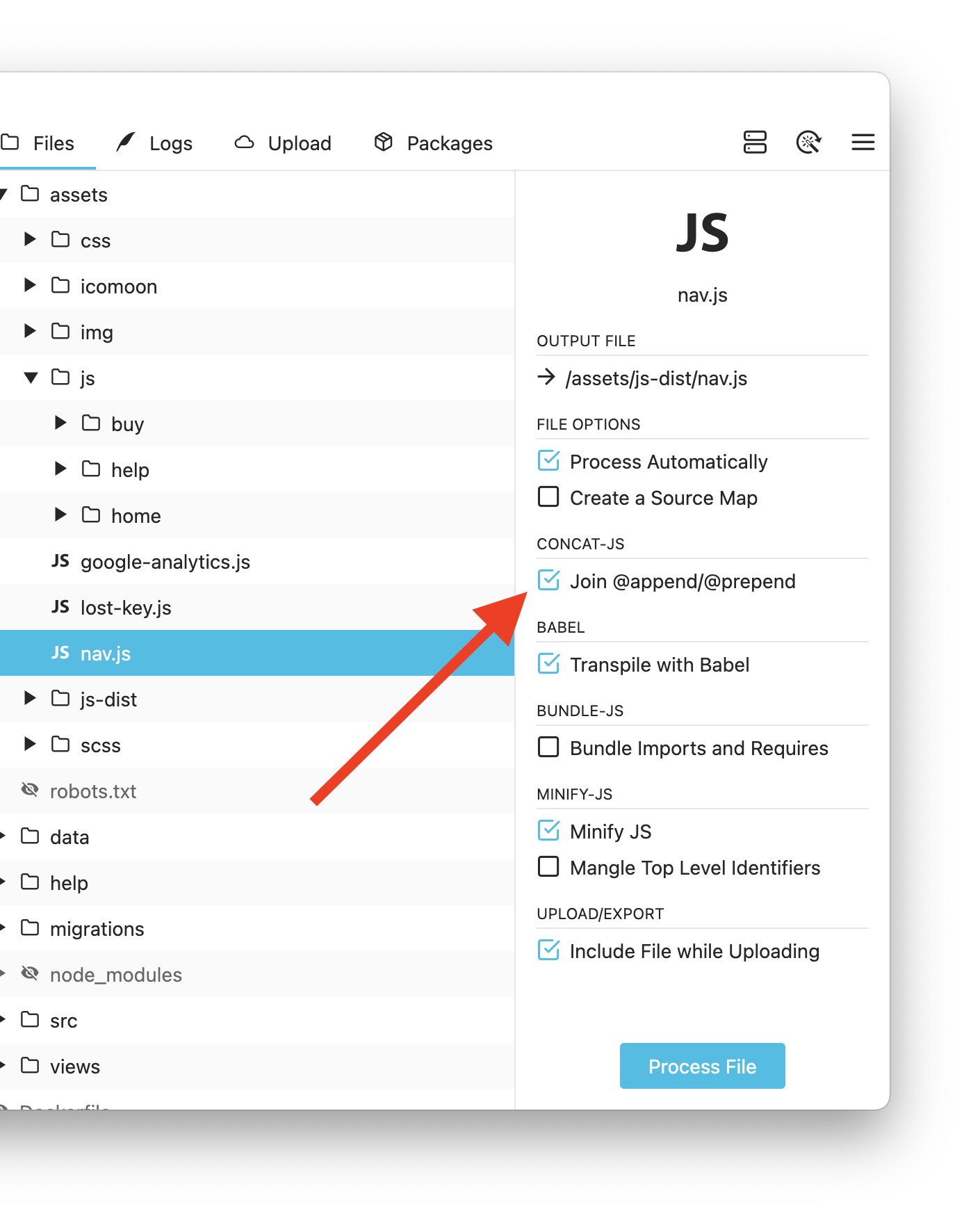
// @prepros-append
Append statements can be used to join a file at the end of another file. For instance if you have a file called a.js
with following append statements.
// @prepros-append first.js
// @prepros-append second.js
Prepros will output a file with the contents of a.js
, first.js
and second.js
combined together in that order.
// @prepros-prepend
Prepend statements can be used to join a file at the beginning of another file. For instance if you have a file called a.js
with following prepend statements.
// @prepros-prepend first.js
// @prepros-prepend second.js
Prepros will output a file with the contents of first.js
, second.js
and a.js
combined together in that order.
You can also use both @prepros-append
and @prepros-prepend
statements in a single file.
Imported Files
Prepros compiles the parent file whenever you edit an imported/required file. Prepros also re-scans imported files whenever you edit a file. Refresh project with CTRL+R or CMD+R to manually re-scan imported files.
For instance if you have a file called a.js
with the following imports.
import './b.js';
require('./c.js');
// @prepros-append d.js
Prepros will process a.js
whenever you edit b.js
, c.js
or d.js
.