Babel
Babel transforms your ES2015+ code into a backwards compatible version of JavaScript. Just enable the “Transpile with Babel” option in the file options sidebar to transform your JavaScript code with Babel.
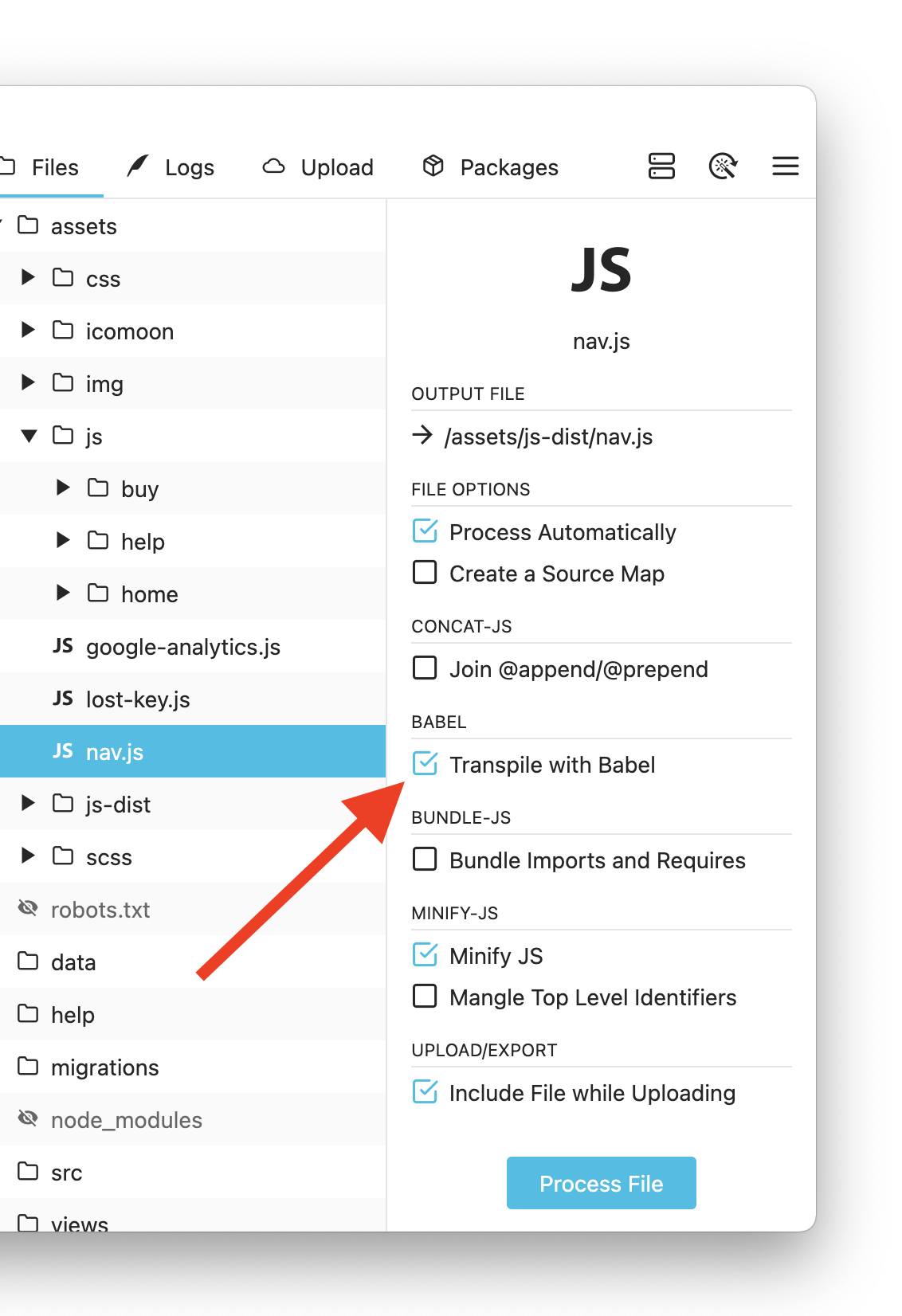
@babel/preset-env
@babel/preset-env
is a smart preset that allows you to use the latest JavaScript features without needing to micromanage which syntax transforms (and optionally, browser polyfills) needed by your target browsers. This makes your life easier and JavaScript bundles smaller. Prepros uses @babel/preset-env
package by default so you don’t have to enable/disable individual babel plugins.
Polyfills
If you enable Insert Polyfills option from Project Settings -> JS Tools -> Babel. Babel inserts import statements containing the necessary core-js
polyfills required to run your JavaScript file on your Target Browsers.
For instance, if your JavaScript uses Map()
and one of your Target Browsers doesn’t support Map()
.
var myMap = new Map();
Babel will insert an import statement containing the necessary core-js
Map()
polyfill.
import 'core-js/modules/es.map';
var myMap = new Map();
You can then use the Bundle Imports and Requires option to bundle the imported polyfill with the output file.
Target Browsers
By default Prepros transpiles your JavaScript to ensure it runs on last 2 versions of all browsers. You can change target browsers from Project Settings -> JS Tools -> Babel to target even older browsers such as IE 11 or IE 10.
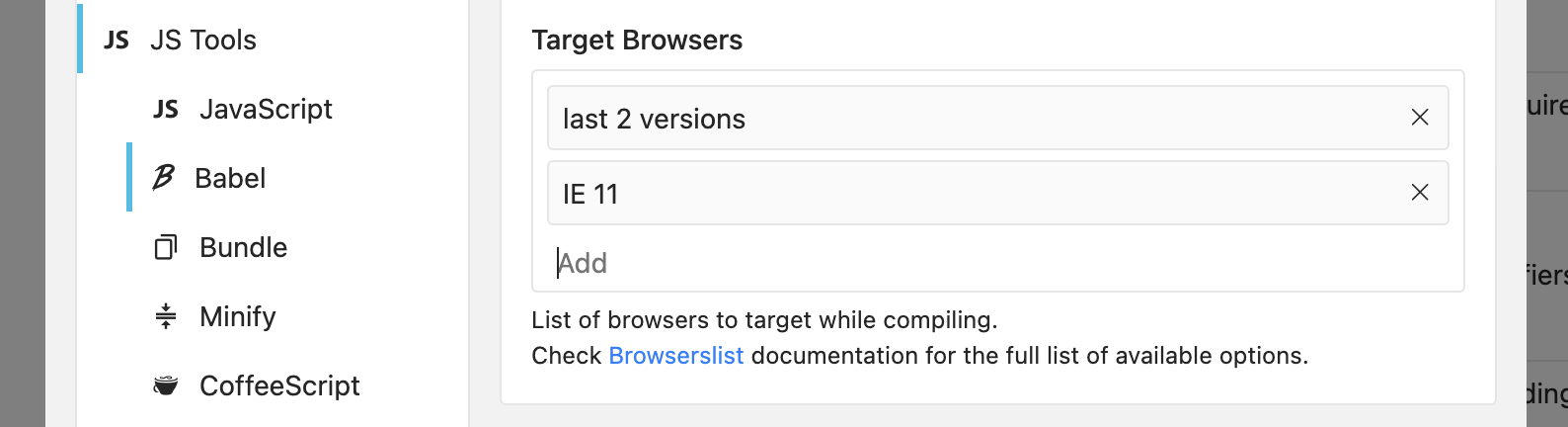
Check Browserlist documentation for the full list of available options.
Built-in Plugins and Presets
Prepros comes bundled with most common presets and plugins required to modernize your JavaScript files.
React and Flow
You can use Babel to transform JSX while building React apps by enabling @babe/preset-react
option from Project Settings -> JS Tools -> Babel. You can also use flow type annotations by enabling the @babe/preset-flow
option.
Experimental Plugins and Presets
@babel/preset-env
transpiles most modern JavaScript features such let
, const
, for of
, async
etc.
These features are standardized by Ecma International, Technical Committee 39. You can use these features by enabling the @babel/preset-env
option.
Prepros also comes bundled with plugins for Stage 2+ proposals which are in the process of being standardized. These experimental features are stable enough to be used. You can enable these plugins from Project Settings -> JS Tools -> Babel.
Custom Plugins and Presets
If you want to use plugins and presets which are not bundled with Prepros you can do that by installing them with NPM into your project folder then adding them to the Custom Plugins and Presets list in Project Settings -> JS Tools -> Babel.
Installing a Plugin with NPM
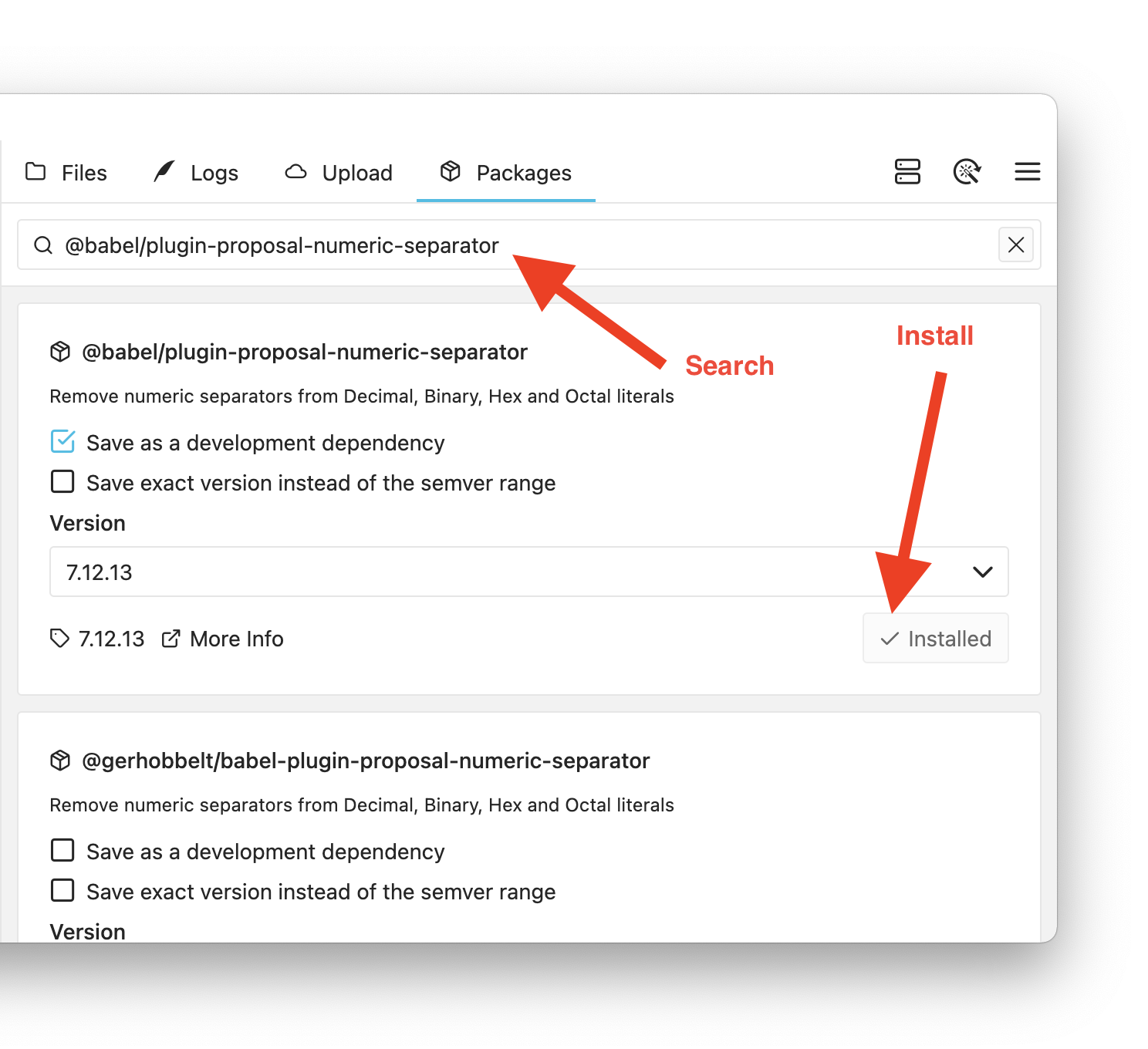
Configuring Custom Plugins and Presets
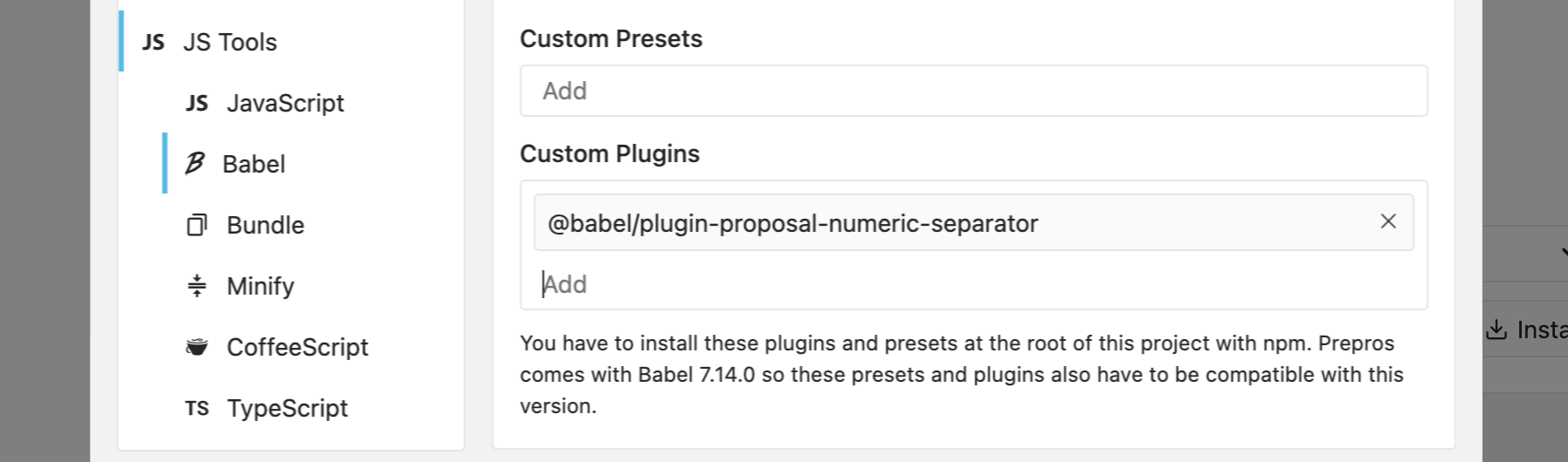
Learn more about Babel from Babel website.